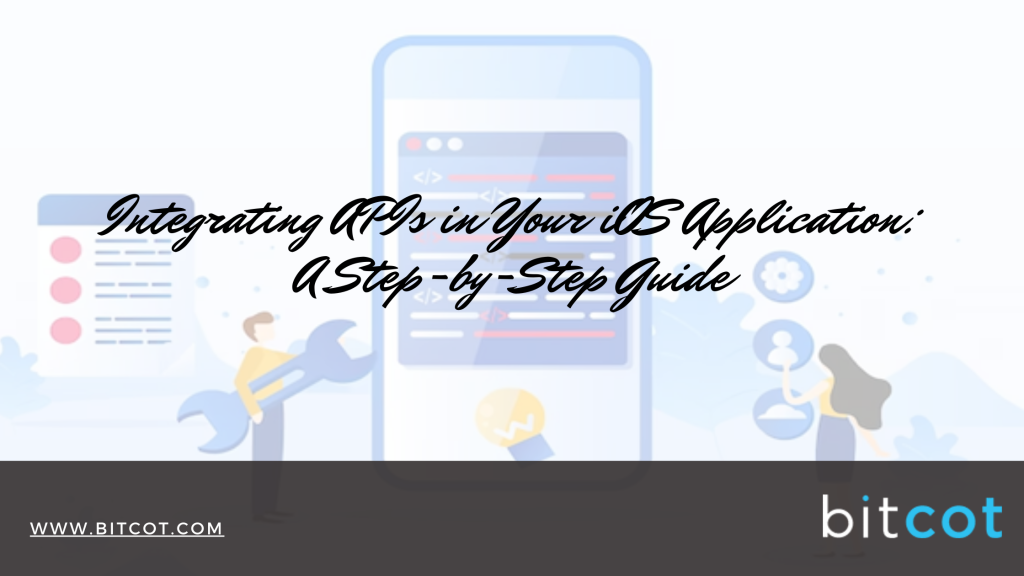
Integrating APIs into your iOS application can significantly enhance its functionality by allowing it to communicate with external services. APIs (Application Programming Interfaces) enable your app to fetch data, interact with other software, and provide dynamic content to users. This step-by-step guide will help you understand the process of integrating APIs into your iOS app, covering everything from setting up your project to handling API responses.
Step 1: Setting Up Your Project
Before you start integrating an API, ensure your Xcode project is ready. Create a new Xcode project or open an existing one where you want to implement API integration. Ensure you have a stable internet connection since you’ll need to install dependencies and possibly test API calls.
Step 2: Choose an API and Obtain API Keys
Choose the API you want to integrate. Popular choices include weather APIs, social media APIs, or custom backend APIs. Once you’ve selected an API, sign up (if required) and obtain your API key. This key is essential for authenticating your requests.
Step 3: Install Alamofire
Alamofire is a popular Swift-based HTTP networking library that simplifies API integration. You can install Alamofire using CocoaPods, Swift Package Manager, or Carthage. Here’s how to install it using CocoaPods:
1. **Install CocoaPods** (if you haven’t already):
“`bash
sudo gem install cocoapods
“`
2. **Initialize CocoaPods in your project directory**:
“`bash
pod init
“`
3. **Add Alamofire to your Podfile**:
“`ruby
pod ‘Alamofire’, ‘~> 5.4’
“`
4. **Install the dependency**:
“`bash
pod install
“`
5. **Open the `.xcworkspace` file** created by CocoaPods:
“`bash
open YourProject.xcworkspace
“`
Step 4: Configure Network Requests
Create a new Swift file in your project, such as `NetworkManager.swift`, to handle all network-related tasks.
“`swift
import Foundation
import Alamofire
class NetworkManager {
static let shared = NetworkManager()
private init() {}
func fetchData(from url: String, completion: @escaping (Result<Data, Error>) -> Void) {
AF.request(url).validate().responseData { response in
switch response.result {
case .success(let data):
completion(.success(data))
case .failure(let error):
completion(.failure(error))
}
}
}
}
“`
Step 5: Make an API Call
Now, you can use the `NetworkManager` to make an API call. For example, let’s fetch data from a sample API endpoint.
“`swift
import UIKit
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
let sampleAPIURL = “https://api.example.com/data”
NetworkManager.shared.fetchData(from: sampleAPIURL) { result in
switch result {
case .success(let data):
self.handleResponseData(data)
case .failure(let error):
print(“Error fetching data: \(error)”)
}
}
}
private func handleResponseData(_ data: Data) {
// Handle your API response data here
// For example, parse JSON data
do {
let json = try JSONSerialization.jsonObject(with: data, options: [])
print(“Response JSON: \(json)”)
} catch {
print(“Error parsing JSON: \(error)”)
}
}
}
“`
Step 6: Handle JSON Data
Most APIs return data in JSON format. You need to parse this JSON data to use it effectively in your application. You can use Swift’s `Codable` protocol for this purpose.
“`swift
struct APIResponse: Codable {
let id: Int
let name: String
let description: String
}
private func handleResponseData(_ data: Data) {
do {
let decoder = JSONDecoder()
let response = try decoder.decode(APIResponse.self, from: data)
print(“ID: \(response.id), Name: \(response.name), Description: \(response.description)”)
} catch {
print(“Error decoding JSON: \(error)”)
}
}
“`
Step 7: Error Handling
Robust error handling is crucial for a smooth user experience. Ensure you provide meaningful feedback to the user in case of network errors or invalid responses.
“`swift
import UIKit
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
let sampleAPIURL = “https://api.example.com/data”
NetworkManager.shared.fetchData(from: sampleAPIURL) { result in
switch result {
case .success(let data):
self.handleResponseData(data)
case .failure(let error):
self.handleError(error)
}
}
}
private func handleResponseData(_ data: Data) {
// Handle your API response data here
do {
let decoder = JSONDecoder()
let response = try decoder.decode(APIResponse.self, from: data)
print(“ID: \(response.id), Name: \(response.name), Description: \(response.description)”)
} catch {
print(“Error decoding JSON: \(error)”)
}
}
private func handleError(_ error: Error) {
// Handle the error appropriately
print(“Network error occurred: \(error)”)
// Show an alert to the user
let alert = UIAlertController(title: “Error”, message: “Failed to load data. Please try again later.”, preferredStyle: .alert)
alert.addAction(UIAlertAction(title: “OK”, style: .default, handler: nil))
self.present(alert, animated: true, completion: nil)
}
}
“`
Step 8: Securing Your API Keys
Never hardcode your API keys directly into your app. Instead, use environment variables or configuration files that are not included in your version control system. For example, you can create a `Config.plist` file to store your API keys securely.
1. **Create `Config.plist`**:
– Right-click on your project in Xcode and select “New File”.
– Choose “Property List” and name it `Config.plist`.
2. **Add Your API Key**:
– Open `Config.plist` and add a new key-value pair for your API key.
3. **Access the API Key in Your Code**:
“`swift
if let path = Bundle.main.path(forResource: “Config”, ofType: “plist”),
let config = NSDictionary(contentsOfFile: path),
let apiKey = config[“API_KEY”] as? String {
print(“API Key: \(apiKey)”)
}
“`
Step 9: Testing Your API Integration
Thoroughly test your API integration to ensure it works correctly under various conditions. Use tools like Postman to test API endpoints independently and make sure your app handles different responses appropriately.
Conclusion
Integrating APIs into your iOS application can significantly enhance its functionality and user experience. Following these steps, you can effectively set up your project, make API calls, handle responses, and ensure robust error handling. Remember to secure your API keys and thoroughly test your integration to provide a smooth experience for your users. With these best practices, you’ll be well on your way to creating powerful and dynamic iOS applications.
Also Read: 6 Reasons On Why Should A Business Choose iOS App Development Platform?